This week, we moved beyond simply “using technology” and started asking ourselves:
Who is included? Who is excluded? And how can we be more critically and empathetically thinking in digital spaces?
From deep musings on digital equity by Maha Bali, to examining our own physical well-being while working online, this week enabled me tothink about the human impacts of technologyâon our minds, bodies, and selves.
đ§ Critical Thinking: Thinking About Thinking
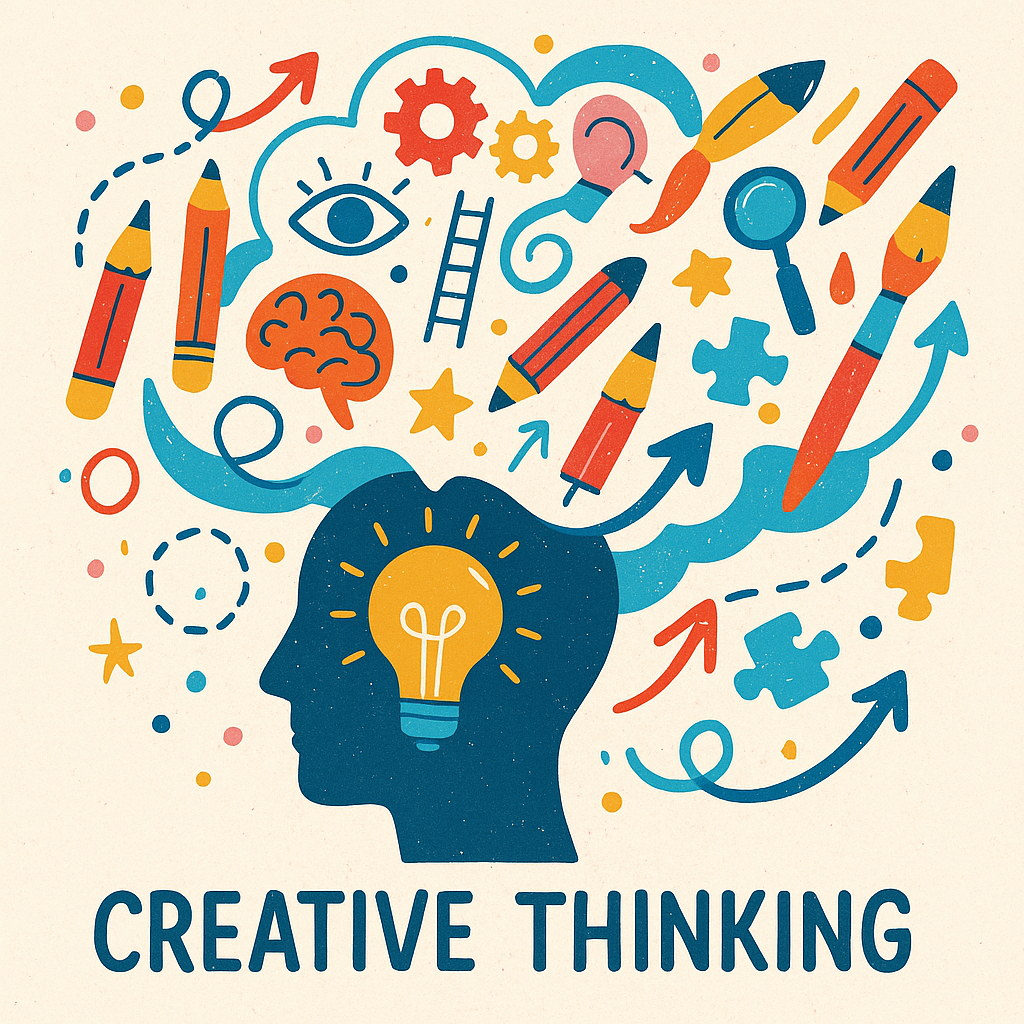
Although Thomas Landâs talk on critical thinking was private, it prompted me to reflect on how I process informationâespecially online. Critical thinking isnât just about spotting logical fallacies. Itâs about:
â Questioning sources
â Understanding context
â Recognizing our own biases
â Staying open to other perspectives
đĄÂ Reflection:
In a time when misinformation and AI-generated content are everywhere, critical thinking is arguably the most important digital skill we can have. I’ve started paying more attention to how I evaluate content I come acrossâespecially when something confirms my existing opinions a bit too perfectly.
đ Equity in Digital Spaces with Maha Bali
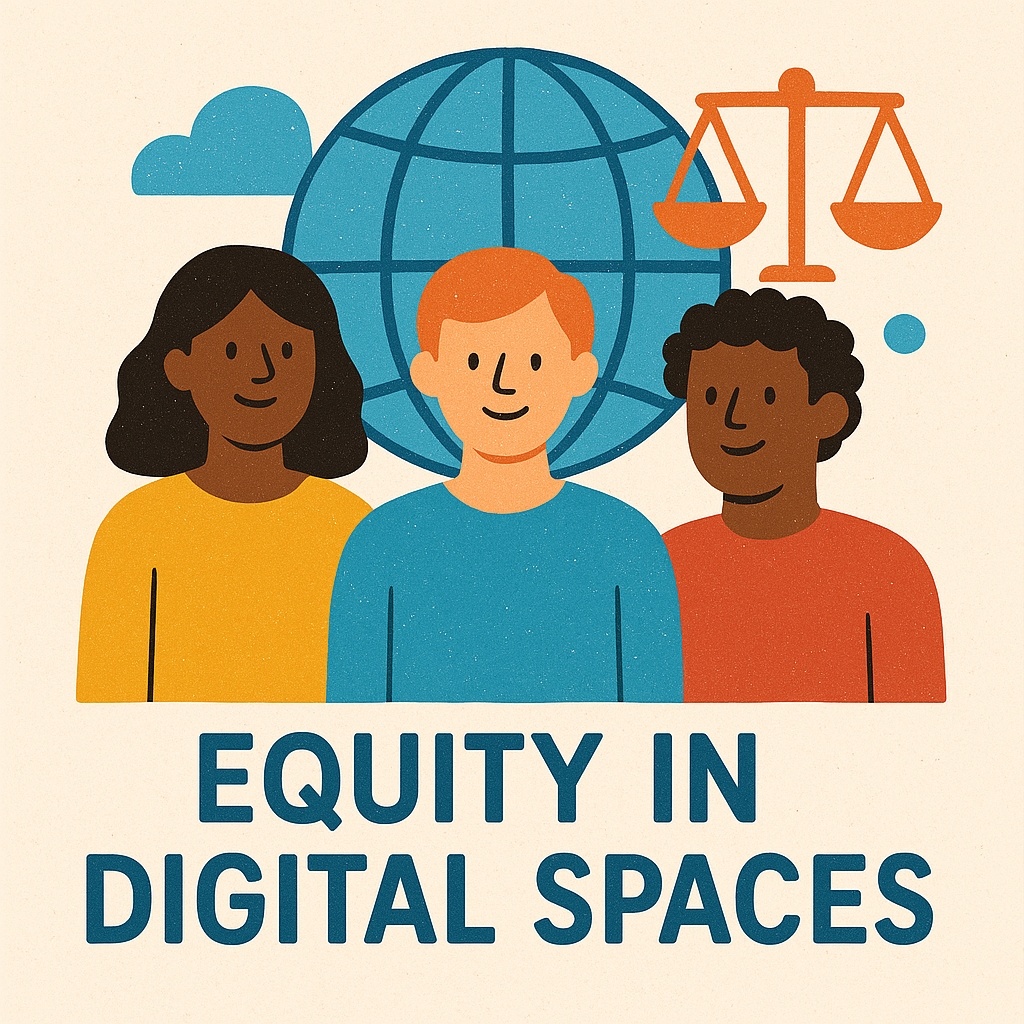
Maha Baliâs talk was a game-changer for me. Her explanation of Nancy Fraserâs frameworkâeconomic, cultural, and political justiceâgave me the language to think about inequality in tech more clearly.
đ Highlights That Stood Out:
- Economic injustice isn’t just about internet or devicesâit’s also about time, labor, and basic needs like food and safety
- Cultural injustice occurs when marginalized voices are missing or misrepresented (e.g., AI systems repeating colonial or stereotypical forms of narrative)
- Political injustice occurs when people are not being included in creating systemsâlike being “invited to a table that’s already been set
đŹ One quote from Maha that stuck with me:
âTechnology is not neutral. It reflects and amplifies the biases of those who create and control it.â
She also suggested frameworks like the 4 I’s of Oppression (ideological, institutional, interpersonal, and internalized) and her clever “Rumi cheese” metaphorâa spicier variation on the Swiss cheese model that shows we need layered protections from different forms of injustice in online life.
I now get it that digital inclusion is not a checklist. It’s listening, redesigning systems, and making space for difference. It also reminded me to ask myself the questions of who created the tools I am usingâWho benefits? Who is harmed?
đş Ergonomics: Caring for the Body Thatâs Always Online
As someone who spends hours at my laptop, I rarely think about the toll on my body until I start feeling neck or wrist pain. This weekâs ergonomics resources were a great wake-up call.
â What I Did:
- Reviewed UVicâs Ergonomics Checklist (PDF)
- Watched several modules on workstation posture, lighting, and computer input devices
- Took stretch breaks during study sessions and adjusted my chair height
đ One key lesson: Micro-adjustments = big difference.
Just changing my screen angle and adding a small pillow behind my lower back relieved a lot of tension.
đĄ Reflection:
Wellness is part of digital literacy. A successful digital existence also has to be a healthy and sustainable one. And I now understand that ergonomics is not just for “office workers”âit’s for all of us who live online.
đ Connecting the Dots
These three topicsâcritical thinking, digital equity, and ergonomicsâmight, at first sight, have very little to do with each other. But taken together, they made me notice something important:
Digital spaces reflect real power relations and personal habits. If we want better results, we must approach them warily, in awe, and with a questioning mind.